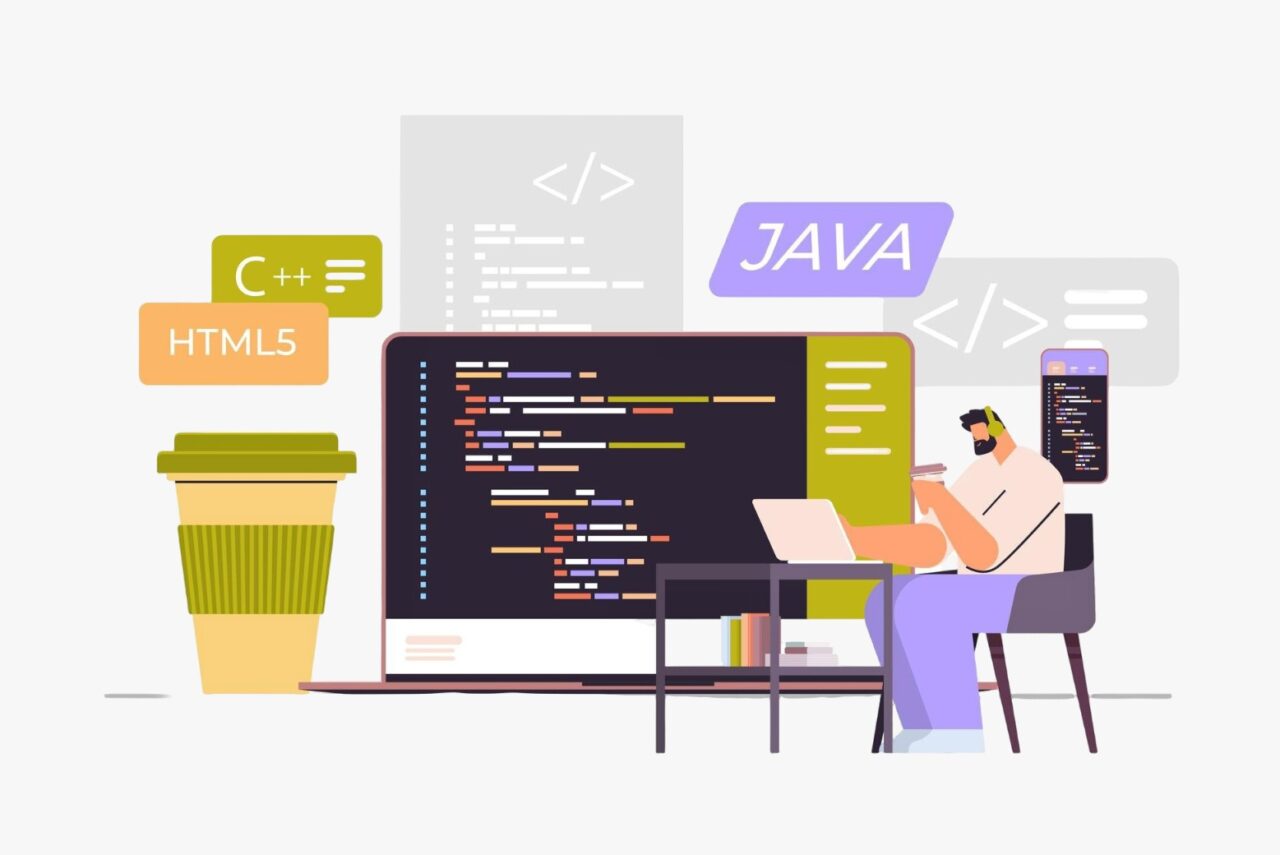
Java fullstack
Instructor
predragacademy
Reviews
Course Overview
1. Introduction to Java
- History and Features of Java
- Java Virtual Machine (JVM), Java Runtime Environment (JRE), and Java Development Kit (JDK)
- Java Development Environment Setup o Basic Syntax and Structure
2. Basic Programming Concepts
- Data Types and Variables
- Operators (Arithmetic, Relational, Logical, etc.)
- Control Statements (if, else, switch, for, while, do-while)
- Basic Input and Output
3. Arrays and Strings
- Single-Dimensional and Multi-Dimensional Arrays
- String Class and String Manipulation
- StringBuilder and StringBuffer
4. Object-Oriented Programming (OOP) Concepts
- Classes and Objects
- Methods and Constructors
- Inheritance (Single, Multilevel, Hierarchical)
- Polymorphism (Method Overloading and Overriding)
- Encapsulation (Access Modifiers)
- Abstraction (Abstract Classes and Interfaces)
5. Exception Handling
- Introduction to Exceptions
- try, catch, finally Blocks
- throw and throws Keywords
- Creating Custom Exceptions
6. Collections Framework
- Introduction to Collections
- List Interface (ArrayList, LinkedList)
- Set Interface (HashSet, LinkedHashSet)
- Map Interface (HashMap)
- Iterators and Enhanced For Loop
7. Java I/O (Input/Output)
- File Handling (File Class, FileReader, FileWriter)
- Byte Streams and Character Streams
8. Threads and Concurrency
- Introduction to Threads
- Creating Threads (Extending Thread Class, Implementing Runnable Interface)
- Thread Life Cycle and States
- Synchronization and Locks
- Concurrency Utilities (Executors, Callable, Future)
9. Java 8 Features
- Lambda Expressions
- Functional Interfaces
10. Advanced Topics
- Generics
- Java Memory Management and Garbage Collection
11. MySQL Database
- Introduction to MySQL
- Setting Up MySQL Server and MySQL Workbench
- Basic SQL Commands: SELECT, INSERT, UPDATE, DELETE
- Database Schema Design: Tables, Keys, Relationships
- Advanced SQL Queries: Joins, Subqueries, Aggregations
- Using MySQL with JDBC: Connecting Java Applications to MySQL
12. JDBC (Java Database Connectivity)
- Overview: Connecting to Databases
- Components: DriverManager, Connection, Statement
- Executing Queries: CRUD Operations, Handling ResultSet
- Transactions: Committing and Rolling Back
13. JSP (JavaServer Pages)
- Basics: Syntax, Directives, and Lifecycle
- JSTL: Core and Formatting Tags
14. Servlets
- Basics: Servlet Lifecycle and Architecture
- HTTP Handling: Methods like doGet() and doPost()
- Session Management: Using HttpSession